Load and Display File Data in Java
- Siah Peih Wee
- Dec 20, 2022
- 2 min read
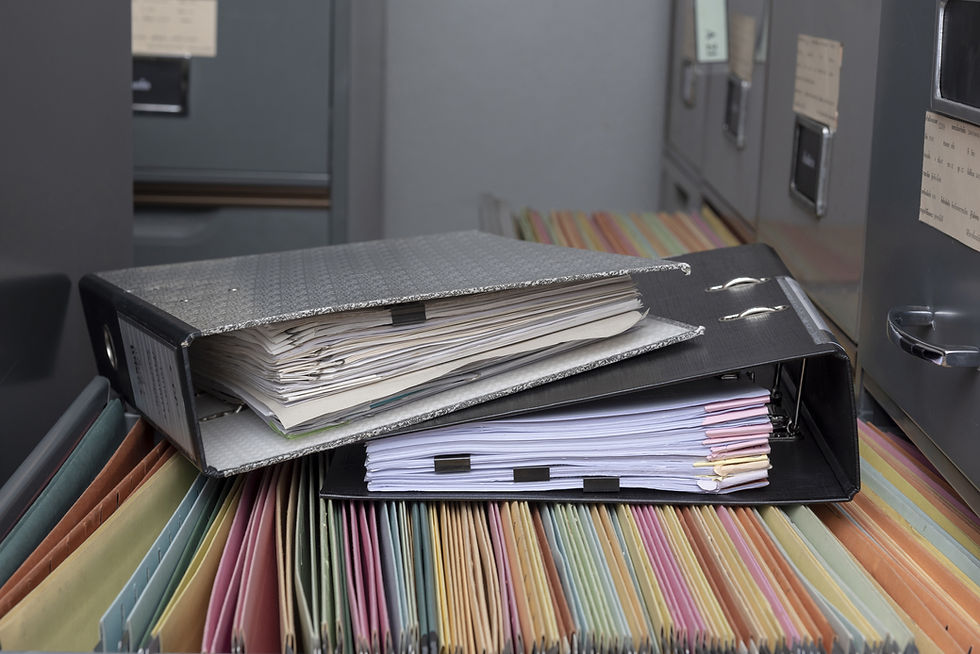
In this tutorial, lets to load file in Java using Repl.it
After you have created an account, click on the button [ + Create ]
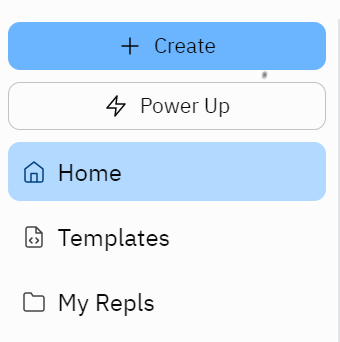
Next, select Java in Template and give your Repl a title before pressing the [ + Create ]
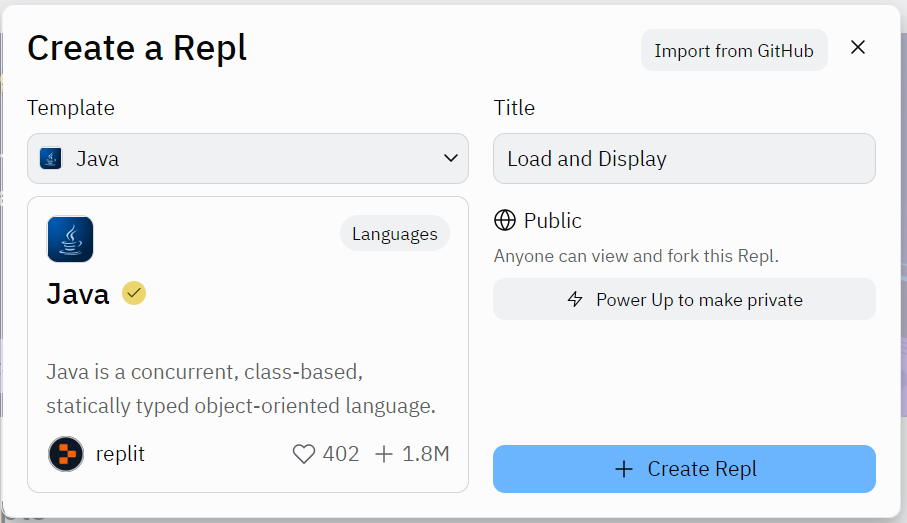
In your Main.java, use Try Catch to load file
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Main
{
public static void main(String[] args)
{
try
{
File fileSave = new File("save.dat");
Scanner objScanner = new Scanner(fileSave);
}
catch (FileNotFoundException e)
{
System.out.println("File Not Found");
}
}
}
When you run the program, you should see File Not Found

Make a save.dat file
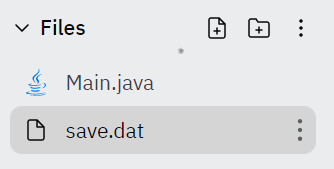
Copy the following data in CSV format and paste into save.dat
#,Name,Type 1,Type 2
1,Bulbasaur,Grass,Poison
2,Ivysaur,Grass,Poison
3,Venusaur,Grass,Poison
4,Charmander,Fire,
5,Charmeleon,Fire,
6,Charizard,Fire,
7,Squirtle,Water,
8,Wartortle,Water,
9,Blastoise,Water,
When you run the program, you won't see "File Not Found" any more. This means the file has been successfully loaded.
After the line that instantiate Scanner.
Scanner objScanner = new Scanner(fileSave);
Add the following codes
int iLines = 0;
while (objScanner.hasNextLine())
{
String sLine = objScanner.nextLine();
iLines++;
System.out.println(sLine);
}
System.out.println("Total Lines Found: "+iLines);
objScanner.close();
You can view the following Repl.it to see it in action
If you want it to display nicely in the JOptionPane, you will need to use JTextArea
JTextArea objTArea = new JTextArea(sMsg);
objTArea.setOpaque(false);
JOptionPane.showMessageDialog(null, objTArea, "Pokemon",
JOptionPane.INFORMATION_MESSAGE);
Each line of data in save.dat, you will need to split using the comma symbol
String[] aCol = sLine.split(",");
Using the For Each loop, you can separate each column using the "\t" or Tab.
int iCol = 0;
for(String sData : aCol)
{
sMsg += sData;
if(iCol == 0) sMsg += " ";
if(iCol > 0) sMsg += "\t";
if(iCol == 1 && sData.length() < 10) sMsg += "\t";
iCol++;
}
Below is how the final code will look like.
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
import javax.swing.JOptionPane;
import javax.swing.JTextArea;
public class Main
{
public static void main(String[] args)
{
try
{
File fileSave = new File("save.dat");
Scanner objScanner = new Scanner(fileSave);
int iLines = 0;
String sMsg = "";
while (objScanner.hasNextLine()) {
String sLine = objScanner.nextLine();
String[] aCol = sLine.split(",");
iLines++;
int iCol = 0;
for(String sData : aCol)
{
sMsg += sData;
if(iCol == 0) sMsg += " ";
if(iCol > 0) sMsg += "\t";
if(iCol == 1 && sData.length() < 10) sMsg += "\t";
iCol++;
}
if(iLines == 1) sMsg += "\n-----------------------------------------------------------------------------------";
sMsg += "\n";
}
sMsg += "-----------------------------------------------------------------------------------\n";
sMsg += "Total Lines Found: "+iLines;
JTextArea objTArea = new JTextArea(sMsg);
objTArea.setOpaque(false);
JOptionPane.showMessageDialog(null, objTArea, "Pokemon", JOptionPane.INFORMATION_MESSAGE);
objScanner.close();
}
catch (FileNotFoundException e)
{
System.out.println("File Not Found");
}
}
}
You can view the following Repl.it to see it in action
ความคิดเห็น