Declaring Variables in Java
- Siah Peih Wee
- May 2, 2022
- 1 min read
Updated: May 6, 2022
Storing Information
Lets look at a scenario. How should you store the following information?
Quest Completion
Player Name
Currency
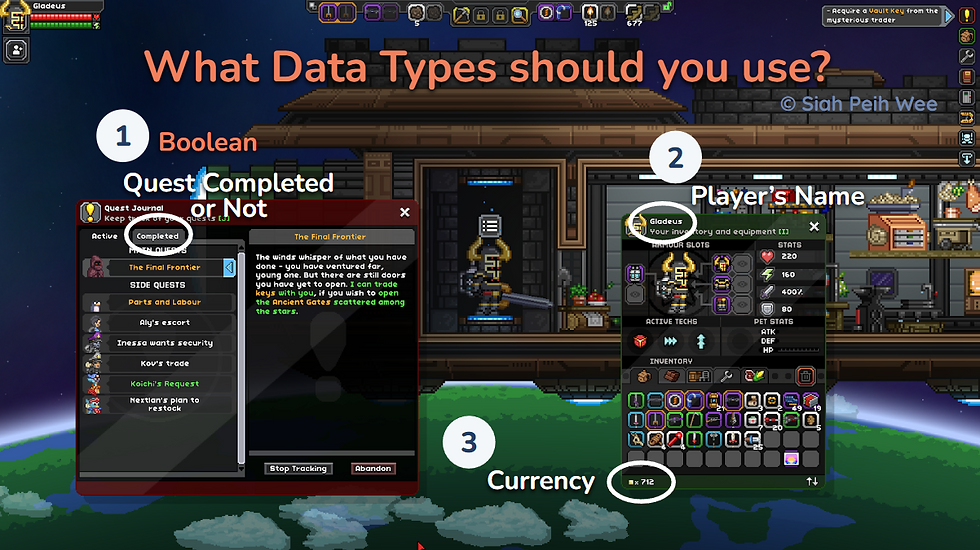
In order to do that, first you must understand what is a variable.
Common Types of Data
String for Text information
Integer for Number information without decimals.
Boolean for logical situation like True or False
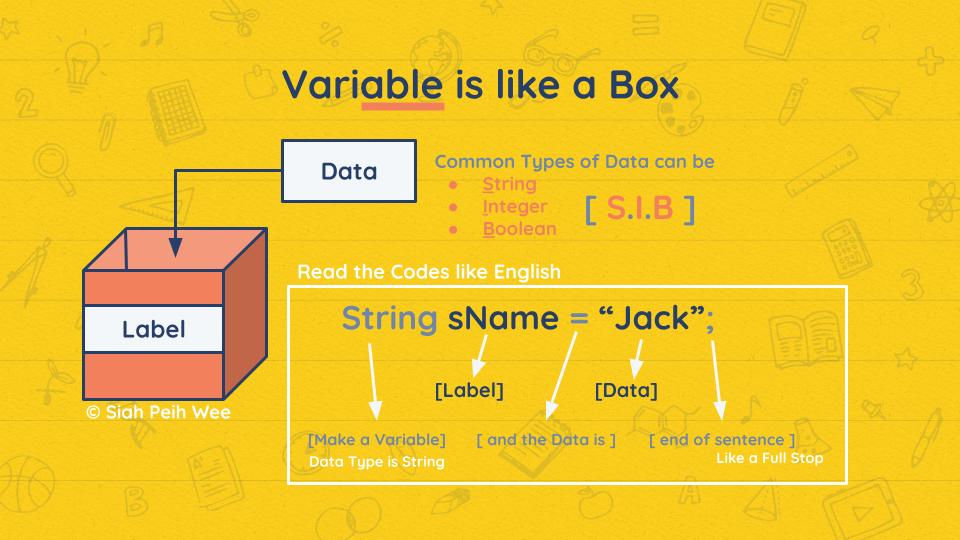
Therefore, in Java. You can declare the variables like the following and print them out.
public class BasicVariables
{
public static void main(String[] args)
{
String sPlayerName = "Gladeus";
int iCurrency = 712;
boolean bCompletedQuest = false;
System.out.println("Player Name: " + sPlayerName);
System.out.println("Currency: " + iCurrency);
System.out.println("Quest Completed: " + bCompletedQuest);
}
}
Data Types
Java is a statically typed language, at compile time its variables & its data type are defined. Therefore, such variables cannot hold other data types after declaration.
So what other data types are there?
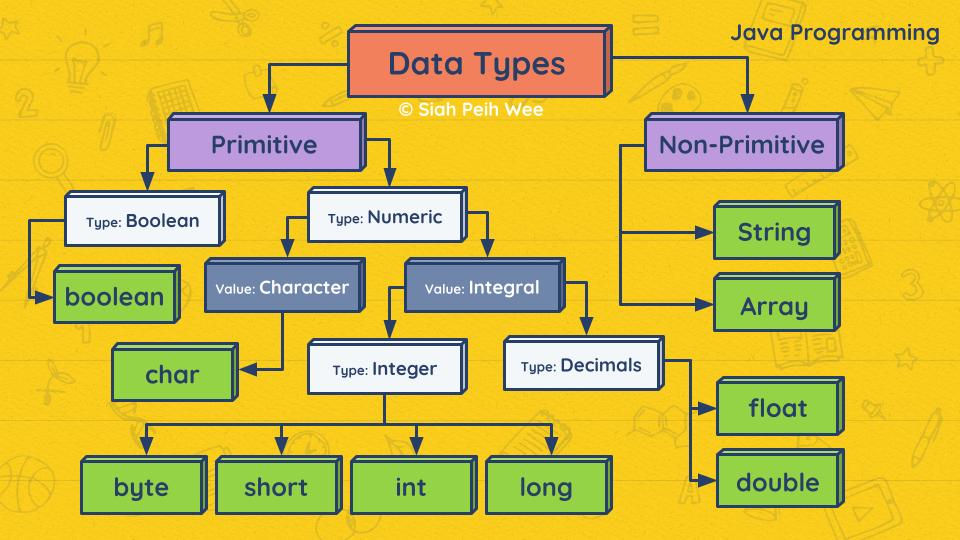
You may read more here
Comments